Assignment No. 03 : Program to accept marks of five courses and compute the result.
- anitadevkar
- Jan 30, 2022
- 1 min read
Updated: Feb 1, 2022
Problem Statement: Write a program to accept marks of five courses and compute the result. If student scores aggregate greater than 75%, then the grade is distinction. If aggregate is 60>= and <75 then the grade is first division. If aggregate is 50>= and <60, then the grade is second division. If aggregate is 40>= and <50 then the grade is third division. If aggregate is < 40 then student is fail.
Program:
#include <stdio.h>
int main()
{
int maths,physics,chemistry,english,computer,total;
float per;
printf("Enter your marks (out of 100)\n");
printf("Enter Maths Marks:");
scanf("%d",&maths);
printf("Enter Physics Marks: ");
scanf("%d",&physics);
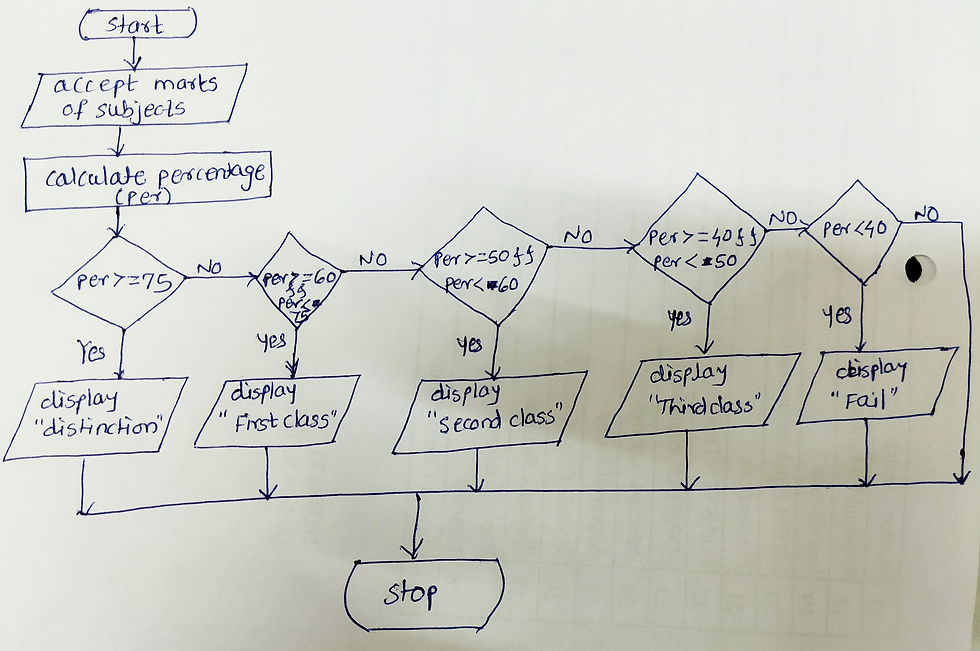
printf("Enter Chemistry Marks: ");
scanf("%d",&chemistry);
printf("Enter English Marks: ");
scanf("%d",&english);
printf("Enter Computer Marks: ");
scanf("%d",&computer);
total = maths+physics+chemistry+english+computer;
per = (total*100)/500;
printf("Total percentage is %0.2f%%\n",per);
if(per>=75)
printf("You have passed with Distinction \n");
else if(per>=60&&per<75)
printf("Your have passed with First Grade\n");
else if(per>=50&&per<60)
printf("Your have passed with Second Grade\n");
else if(per>=40&&per<50)
printf("Your have passed with Third Grade\n");
else if(per<40)
printf("You are Failed\n");
return 0;
}
Output:
Test case-1:
Enter your marks (out of 100)
Enter Maths Marks: 79
Enter Physics Marks: 76
Enter Chemistry Marks: 90
Enter English Marks: 78
Enter Computer Marks: 80
Total percentage is 80.00%
You have passed with Distinction
Test case-2:
Enter your marks (out of 100)
Enter Maths Marks:56
Enter Physics Marks: 55
Enter Chemistry Marks: 60
Enter English Marks: 45
Enter Computer Marks: 59
Total percentage is 55.00%
Your have passed with Second Grade
Test case-3:
Enter your marks (out of 100)
Enter Maths Marks:35
Enter Physics Marks: 39
Enter Chemistry Marks: 40
Enter English Marks: 34
Enter Computer Marks: 37
Total percentage is 37.00%
You are Failed
Steps:
Accept input from user as five subject Marks
Calculate percentage using formula.
Check the condition and accordingly print the message.
Comments