Problem Statement: A hotel has a pricing policy as follows:-
Stay for 2 person : 2500Rs. per night
Stay for 3 person : 3500Rs. per night
Stay for 4 person : 4500Rs. per night
Additional person : 1000Rs. per person per night
If the customer is staying on company business tour, there is a 20% discount. Take the number of people, number of night staying, if it's business tour or not as input from user. Write a program to calculate and print the cost of the room.
Program:
#include <stdio.h>
int main()
{
int no_people,no_night,is_business;
float cost,total_cost;
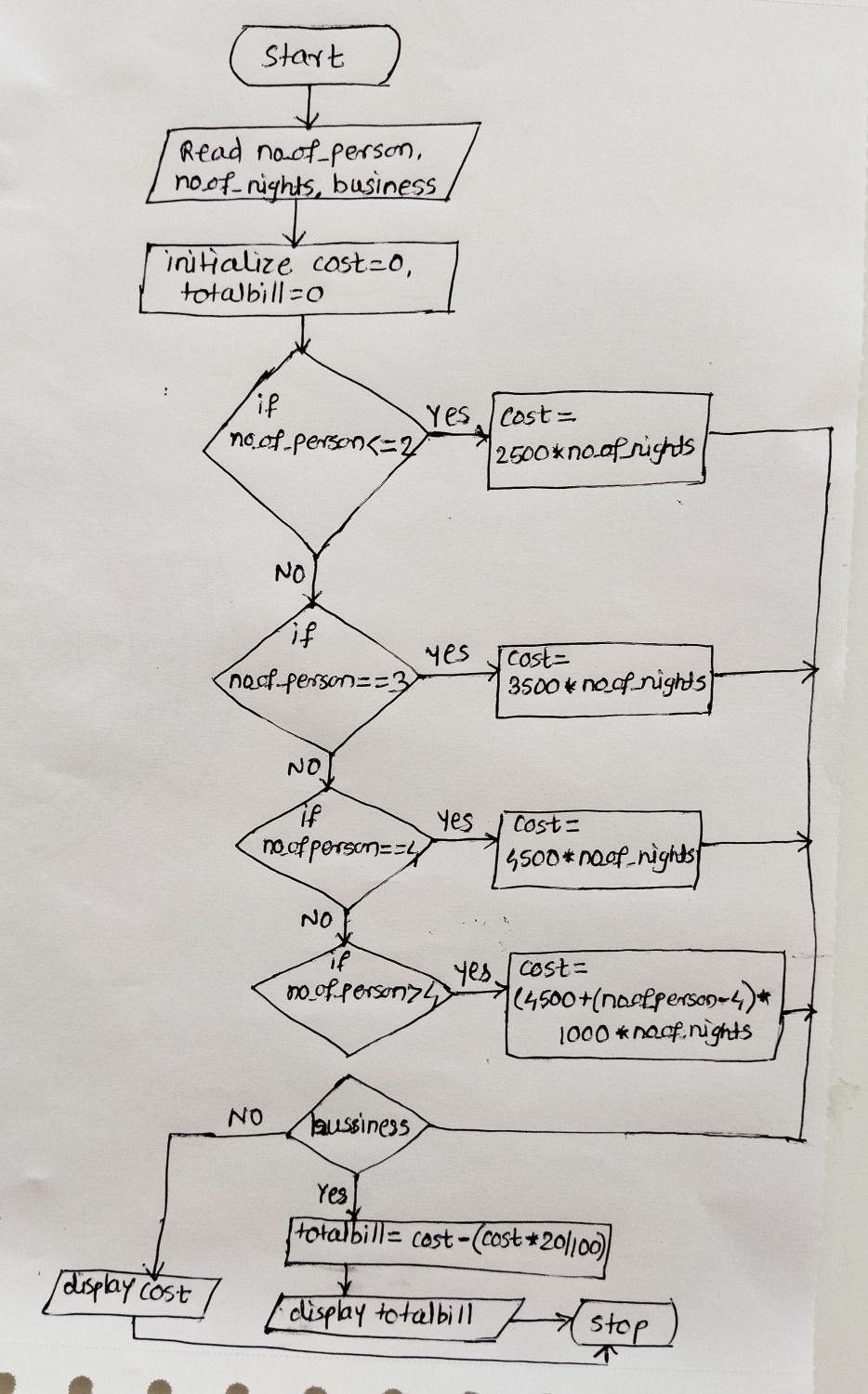
printf("Enter the no of people staying in hotel\t");
scanf("%d",&no_people);
printf("\nEnter the no of days staying in hotel\t");
scanf("%d",&no_night);
printf("\nPress(1) if he/she is on business tour, else(0)\t");
scanf("%d",&is_business);
if(no_people<=2)
cost=2500*no_night;
else if(no_people==3)
cost=3500*no_night;
else if(no_people==4)
cost=4500*no_night;
else if(no_people>4)
cost=(4500+(no_people-4)*1000)*no_night;
if(is_business==1)
{
// total_cost=cost-cost/5;
total_cost=cost-(cost*20/100);
printf("\nTotal Cost payable after getting discount is Rs= %.2f\t",total_cost);
}
else
printf("\nTotal Cost payable is Rs= %.2f\t",cost);
return 0;
}
Output:
Test case-1:
Enter the no of people staying in hotel 2
Enter the no of days staying in hotel 2
Press(1) if he/she is on business tour, else(0) 1
Total Cost payable after getting discount is Rs= 4000.00
Test case-2:
Enter the no of people staying in hotel 6
Enter the no of days staying in hotel 2
Press(1) if he/she is on business tour, else(0) 1
Total Cost payable after getting discount is Rs= 10400.00
Test case-3:
Enter the no of people staying in hotel 3
Enter the no of days staying in hotel 2
Press(1) if he/she is on business tour, else(0) 0
Total Cost payable is Rs= 7000.00
Steps:
Accept input from user as no_person,no_nights,is_business
depending on no of person staying for no of days in hotel (condition) calculate cost.
Check if person is staying for business purpose if yes then give discount as 20% on total cost.
finally print the cost of the room.
Kommentare